The Skype Developer Platform for Web ("Skype Web SDK") is a set of JavaScript Web APIs and HTML controls that enable you to build web experiences that seamlessly integrate a wide variety of real-time collaboration models leveraging Skype for Business services and the larger Skype network. It provides support for multiple core collaboration services like presence, chat, audio, and video, enabling web experiences across a broad spectrum of users, platforms, and devices.
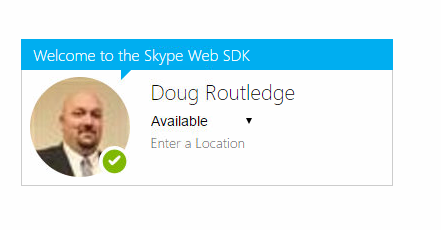
Today's Topic : The AudioService
The AudioServer component of the Skype Web SDK conversation is what you would expect. It is like a telephone component. It represents 2 way audio between 2 participants. Whether you are the caller or the recipient the AudioService methods are the same.
Making a Call
In order to make a call we first need to define a variable to hold the conversation, and define who we want to call.
var conversation = application.conversationsManager.getConversation('sip:XXXX'); // or var conversation = application.conversationsManager.getConversation('tel:+XXXX');
Then we need to start the conversation.
conversation.audioService.start();
The Call State
Once the conversation begins we need to monitor the state of the conversation. The reason for this is similar to how you use a phone. You don't start speaking while it is ringing and expect the other part to be able to hear you. The states are pretty simple and defined like this.
Created - The state when the conversation was created.
Connecting - The state while the conversation is being created.
Connected - The state when the call is connected and both parties are able to participate with voice.
Disconnected - The state when the call is disconnected, when a party has left.
So how do we react to certain changes in the Audio Modality State?
conversation.selfParticipant.audio.state.when('Connected', function () {
//our code here
});
There are 2 ways to end an audio call. You can call the stop or leave functions to accomplish this. Stop would leave the other modalities in place, but leave will close all modalities.
conversation.leave().then(function () {
// successfully left the conversation
}, function (error) {
// error
});
// OR
conversation.audioService.stop().then(function () {
// successfully stopped audio
}, function (error) {
console.log("Failed to stop audio: " + error);
});
Here is how we could sum up the entire AudioService in one code block.
var conversation = application.conversationsManager.getConversation('sip:XXXX');
OR
var conversation = application.conversationsManager.getConversation('tel:+XXXX');
conversation.selfParticipant.audio.state.when('Connected', function () {
console.log('Connected to audio call');
});
conversation.state.changed(function (newValue, reason, oldValue) {
console.log('Conversation state changed from', oldValue, 'to', newValue);
});
conversation.participants.added(function (participant) {
console.log('Participant:', participant.displayName(), 'has been added to the conversation');
});
conversation.audioService.start().then(function() {
console.log('The call has been started successfully');
}, function (error) {
console.log('An error occured starting the call', error);
});
Doug Routledge, C# Lync, Skype for Business, SQL, Exchange, UC,
Full Stack Developer BridgeOC Bridge Operator Console Twitter - @droutledge @ndbridge |
nice post
ReplyDeleteHi Doug, Could you please explain, how to generate a Conference (meeting) URI to schedule a meeting using Web SDK without using SfB native client or outlook?
ReplyDeleteThanks,
H
If you are looking to schedule one you can view this post http://bridgeoc.blogspot.com/2017/08/skype-web-sdk-part-xviiii.html
Delete